Stuck on a Coursera “Introduction to Programming with MATLAB” assignment? Get help without spoiling the learning process! This blog post offers guidance and explanations for concepts covered in the course, not just straight-up answers. Strengthen your programming foundation with MATLAB!
This course is perfect for beginners with no programming experience. MATLAB is a user-friendly language ideal for engineers and other professionals because it’s powerful for numerical problems. Even complex problems can be solved with concise MATLAB code, compared to more general-purpose languages.
MATLAB is widely used across science, engineering, finance, and more, making it a valuable skill for many careers.
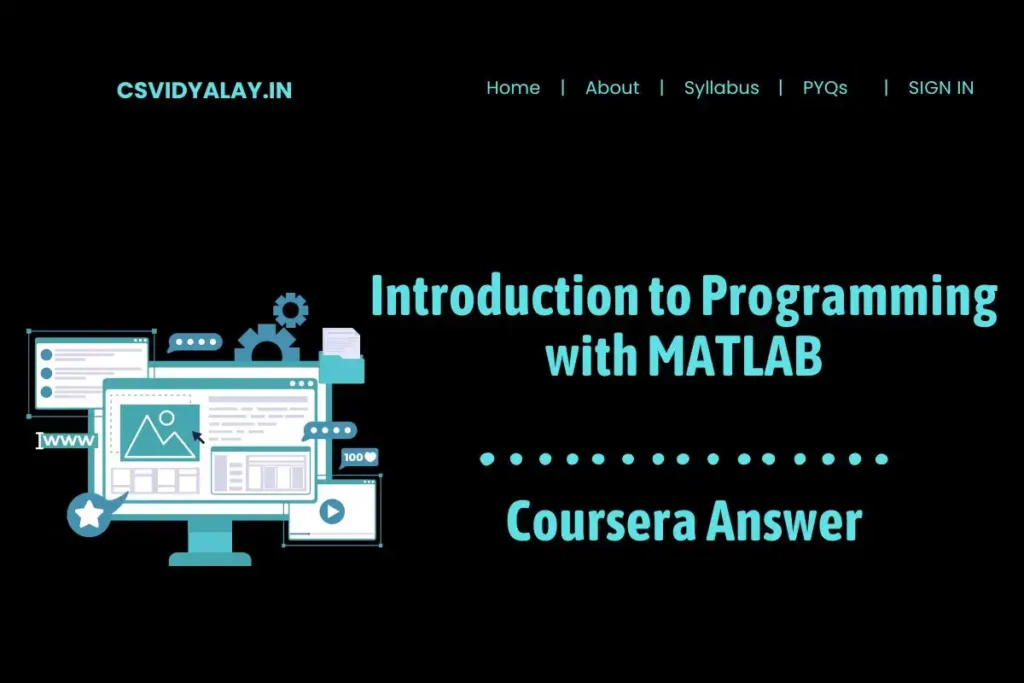
There are 9 modules in the course “Introduction to Programming with MATLAB”
- Course Pages
- The MATLAB Environment
- Matrices and Operators
- Functions
- Programmer’s Toolbox
- Selection
- Loops
- Data Types
- File Input/Output
Contents
- 1 Week 1 – Introduction to Programming with MATLAB
- 2 Week 2 – Introduction to Programming with MATLAB
- 3 Week 3 – Introduction to Programming with MATLAB
- 4 Week 4 – Introduction to Programming with MATLAB
- 5 Week 5 – Introduction to Programming with MATLAB
- 6 Week 6 – Introduction to Programming with MATLAB
- 7 Week 7 – Introduction to Programming with MATLAB
- 8 Week 8 – Introduction to Programming with MATLAB
- 9 Week 9 – Introduction to Programming with MATLAB
Week 1 – Introduction to Programming with MATLAB
No Assignments or Quiz
Week 2 – Introduction to Programming with MATLAB
Week 2 Quiz
No quiz
Week 2 Assignments
Assignment: MATLAB Calculation Code
principal = 1000;
alpha = 0.1;
debt = principal * (1 + alpha) ^ 2;
disp(debt);
Assignment: Lesson 1 Wrap-up Code
time = 9.58 / 3600;
distance = 0.1;
hundred = distance / time;
distance = 42.195;
time = 2 + 1 / 60 + 39 / 3600;
marathon = distance / time;
Week 3 – Introduction to Programming with MATLAB
Week 3 Quiz
1. The value of a after this command: a = [0; 0; 1]’ * [1 2 3; 4 5 6; 7 8 9]
- [3 ; 6 ; 9]
- [7, 8 , 9]
- [3 , 6 , 9]
- [7 ; 8; 9]
2. What will be the value of s after this command: A = [1:4; -2:2:5; 3 1 0 -1]; s = A(end-1,end-1);
- 0
- 2
- We get an error message.
- -1
3. Which of the following is a valid variable name?
- All of the options above are invalid.
- 5x2x3x
- I_Hate_This_Quiz_So_Much
- MATLAB_Rulez!
4. After this command: v = 111:-11:1; how many elements will v have?
- 0
- 10
- 11
- 1
5. In MATLAB, operators work on:
- arguments
- a tight schedule
- associations
- operands
6. The variable t after these commands v = 1:3:33; t = v(end) will be:
- 33
- 32
- 31
- None of the above.
7. The variable t after these commands t = (16:-4:1) ‘ .^ 2 will be
- a 4-element vector
- a 4×4 matrix
- a scalar
- None of the above. MATLAB will print an error message.
8. The value of p after this command: A = [1:4; 5:8; 9:12]; p = size(A);
- will be 12
- will be a 3-by-4 matrix
- will be a 2-element vector
- 4
9. Matrix multiplication works if its two operands
- are vectors; specifically, the first one is a column vector and the second one is a row vector of any length.
- are scalars.
- are square matrices of the same size.
- The first and second options are correct.
- The second and third options are correct.
- All of the options are correct.
10. Array multiplication works if the two operands
- are vectors.
- have the same inner dimensions.
- have the same outer dimension.
- are square matrices of the same size.
Week 3 Assignments:
Assignment: Colon Operator Code:
odds = 1:2:100;
evens = 100:-2:1;
Assignment: Matrix Indexing Code:
A = [1:5; 6:10; 11:15; 16:20];
v = A(:, 2);
A(end, :) = 0;
Assignment: Matrix Arithmetic Code:
A = [1:5; 6:10; 11:15; 16:20];
A1 = ones(1, size(A, 1));
B1 = ones(size(A, 2), 1);
result = A1 * A * B1 ;
Week 4 – Introduction to Programming with MATLAB
Week 4 Quiz:
No Quiz
Week 4 Assignments:
Assignment: A Simple Function Code
function a = tri_area(b, h)
a = 0.5 * b * h;
end
Assignment: Multiple Outputs Code
function [a, b, c, d] = corners(M)
a = M(1, 1);
b = M(1, end);
c = M(end, 1);
d = M(end, end)
end
Assignment: Lesson 3 Wrap-up Code
function fare = taxi_fare(distance, time)
fare = 5 + 2 * (ceil(distance) - 1) + 0.25 * ceil(time);
end
Week 5 – Introduction to Programming with MATLAB
Week 5 Quiz
No quiz
Week 5 Assignments:
Assignment: Built-in functions Code
function [max_min_diff, total_max_min_diff] = minimax(M)
max_rows = max(M');
min_rows = min(M');
max_min_diff = max_rows - min_rows;
total_max_min_diff = max(max_rows) - min(min_rows);
end
Assignment: Lesson 4 Wrap-up Code
function M = trio(n, m)
M = ones(3 * n, m);
M(n + 1 : 2 * n, :) = 2 * ones(n, m);
M(2 * n + 1 : end, :) = 3 * ones(n, m);
end
Week 6 – Introduction to Programming with MATLAB
Week 6 Quiz:
No quiz
Week 6 Assignments:
Assignment: If-statement practice Code
function output = picker(condition, input1, input2)
if condition
output = input1;
else
output = input2;
end
end
Assignment: More practice Code
function admit = eligible(verbal, quant)
admit = mean([verbal quant]) >= 92 && verbal > 88 && quant > 88;
end
Assignment: nargin Code
function too_young = under_age(age, limit)
if nargin == 1
limit = 21;
end
too_young = age < limit;
end
Assignment: Lesson 5 Wrap-up Code
function valid = valid_date(y,m,d)
if nargin == 3
if ~isscalar(y) || y < 1 || y ~= fix(y)
valid = false;
return
elseif ~isscalar(m) || m < 1 || m ~= fix(m)
valid = false;
return
elseif ~isscalar(d) || d < 1 || d ~= fix(d)
valid = false;
return
end
end
a=y/4;b=y/400;c=y/100;
M1 = [1 3 5 7 8 10 12];
M2 = [4,6,9,11];
F1 = (1:29);
F2 = (1:28);
D1 = (1:31);
D2=(1:30);
if a ~= fix(a) || (b ~= fix(b) && c == fix(c))
if ismember(m,M1) && ismember(d,D1)
valid = true;
elseif ismember(m,M2) && ismember(d,D2)
valid = true;
elseif m==2 && ismember(d,F2)
valid = true;
else
valid = false;
end
elseif a == fix(a) || b == fix(b)
if ismember(m,M1) && ismember(d,D1)
valid = true;
elseif ismember(m,M2) && ismember(d,D2)
valid = true;
elseif m==2 && ismember(d,F1)
valid = true;
else
valid = false;
end
end
end
Week 7 – Introduction to Programming with MATLAB
Week 7 Quiz
No quiz
Week 7 Assignments
Assignment: for-loop practice Code
function s = halfsum(M)
[n, m] = size(M);
s = 0;
for row = 1:n
for column = row:m
s = s + M(row, column);
end
end
end
Assignment: while-loop practice Code
function number = next_prime(number)
while ~isprime(number + 1)
number = number + 1;
end
number = number + 1;
end
Assignment: Logical Indexing Code
function count = freezing(temperatures)
count = sum(temperatures < 32);
end
Assignment: Lesson 6 Wrap-up Code
function [summa, index] = max_sum(v, n)
[~, len] = size(v);
summa = -inf;
if n > len
summa = 0;
end
index = -1;
for i = 1:(len - n + 1)
s = sum(v(i : i + n - 1));
if s > summa
summa = s;
index = i;
end
end
end
Week 8 – Introduction to Programming with MATLAB
Week 8 Quiz
No quiz
Week 8 Assignments:
Assignment: Character Vectors Code
function coded = caesar(sequence, shift)
sequence = double(sequence);
sequence = sequence + shift;
coded = char(loop(32, 126, sequence));
end
function val = loop(start, stop, val)
r = stop - start + 1;
val = mod(val - start + r, r) + start;
end
Assignment: Using Cell Arrays Code
function matrix = sparse2matrix(sparse_matrix)
dimensions = cell2mat(sparse_matrix(1));
n = dimensions(1); m = dimensions(2);
default = cell2mat(sparse_matrix(2));
matrix = default * ones(n, m);
[~, len] = size(sparse_matrix);
for i = 3:len
element = cell2mat(sparse_matrix(i));
matrix(element(1), element(2)) = element(3);
end
end
Week 9 – Introduction to Programming with MATLAB
Week 9 Quiz
No quiz
Week 9 Assignments:
Assignment: Excel Files Code
function distance = get_distance(x,y)
persistent raw;
if isempty(raw)
[~, ~, raw] = xlsread('Distances.xlsx');
end
state1_index = state2index(raw, x);
state2_index = state2index(raw, y);
if state1_index > 1 && state2_index > 1
distance = cell2mat(raw(state1_index, state2_index));
else
distance = -1;
end
end
function index = state2index(raw, state)
[~, states] = size(raw);
index = -1;
for i = 2:states
if strcmp(cell2mat(raw(1, i)), state)
index = i;
end
end
end
Assignment: Text Files Code
function charnum = char_counter(file_name, character)
file = fopen(file_name, 'rt');
if file < 0 || ~ischar(character)
charnum = -1;
return;
end
charnum = 0;
line = fgets(file);
while ischar(line)
charnum = charnum + frequency(line, character);
line = fgets(file);
end
end
function count = frequency(line, character)
count = sum(line == character);
end
Assignment: Saddle Points Code
function indices = saddle(M)
indices = zeros(0, 2);
[rows, columns] = size(M);
for row = 1:rows
for column = 1 : columns
point = M(row, column);
if isGreaterThan(point, M(row, :)) && isSmallerThan(point, M(:, column))
indices(end + 1, :) = [row, column]; %#ok<AGROW>
end
end
end
end
function predicate = isGreaterThan(point, vector)
predicate = sum(point >= vector) == length(vector);
end
function predicate = isSmallerThan(point, vector)
predicate = sum(point <= vector) == length(vector);
end
Assignment: Image Blur Code
function [output] = blur(A, w)
[rows, columns] = size(A);
B = nan(size(A) + 2 * w);
B(w + 1 : end - w, w + 1 : end - w) = A;
output = 0 * A;
for i = w + 1 : rows + w
for j = w + 1 : columns + w
tmp = B(i - w : i + w, j - w : j + w);
output(i - w, j - w) = mean(tmp(~isnan(tmp)));
end
end
end
Assignment: Echo Generator Code
function output = echo_gen(in,fs,delay,gain)
samples = round(fs * delay) ;
ds = floor(samples);
signal = zeros(length(in) + ds, 1);
signal(1:length(in)) = in;
echo_signal = zeros(length(in) + ds, 1);
echo_signal(ds + (1:length(in*gain))) = in * gain;
output = signal + echo_signal;
p = max(abs(output));
if p > 1
output = output ./ p;
end
end
You may like:
Google and the Department of Defense Build AI-Powered Microscope to Aid Cancer Detection