Learn Python programming from scratch with this blog post exploring Coursera’s Crash Course on Python Solutions. Discover the benefits of Python for IT careers, understand fundamental syntax, write simple programs, and solve a complex programming problem – all with this comprehensive guide.
Are you interested in learning Python? This course is a great place to start, even if you have no prior programming experience. You’ll learn the basics of Python programming, including the fundamental building blocks and common structures used to write simple programs. By the end of the course, you’ll be able to:
- Understand the advantages of programming in IT careers
- Grasp the core syntax of Python
- Experiment with various code editors and development environments
- Write your own Python programs
- See how programming concepts work together
- Solve a complex programming problem using your newfound knowledge
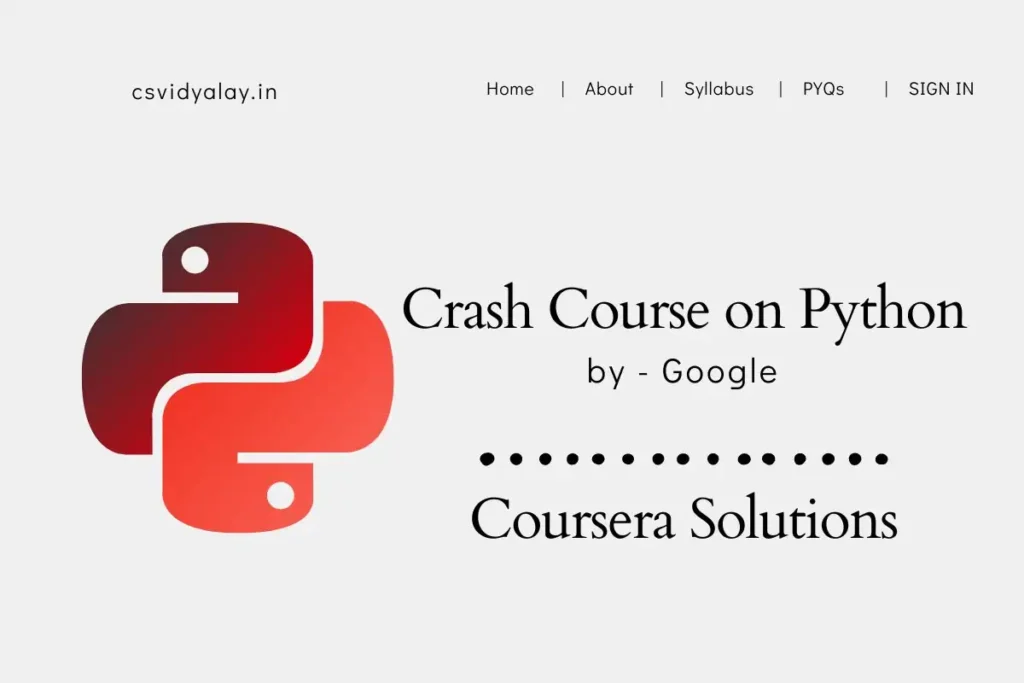
There are six modules in the course “Crash Course on Python”
- Hello Python!
- Basic Python Syntax
- Loops
- Strings, Lists and Dictionaries
- Object Oriented Programming (Optional)
- Final Project
Contents
Week 1 – Crash Course on Python
Week 1 Quiz – Crash Course on Python
1. What is a computer program?
- The syntax and semantics of a programming language.
- Step-by-step instructions on how to complete a set of tasks, to be executed by a computer.
- A file that gets printed by the Python interpreter.
- The overview of what the computer will have to do to solve an automation problem.
2. What is a function?
- The task a program is written to accomplish
- The beginning of a program defining who wrote it and why
- A document describing a software project
- A reusable block of code that performs a specific task
3. What is automation?
- The inputs and outputs of a program
- The process of replacing a manual step with an automated step
- The rules of a programming language
- The process of designing a solution to a problem
4. What is the term for the set of rules for how statements are constructed in a programming language?
- Semantics
- Grammar
- Syntax
- Format
5. Which of the following are characteristics of the Python language? Select all that apply.
- Python is cross-platform compatible
- Python is used in a wide variety of applications
- Python has many platform-specific tools, like Bash or Powershell
- Python is an object-oriented language not fit for general-purpose scripting
6. Which Python function will output text, or other value, to the screen?
- echo
- print()
- output()
- console.out
7. What should be the output of the expression below?
print((9-3)/(2*(1+2)))
- 1.0
- 0.28
- 49.0
- 19.36
8. In one year, if there are 365 days, with 24 hours in a day, , write a program to calculate the number of hours in a year. Print the result on the screen. Note: Your result should be in the format of just a number, not a sentence.
day = 365
hours = 24
result = day * hours
print(result)
9. Use Python to calculate how many different passwords can be formed with 3 lower case English letters (excludes any character not found in the English alphabet). For a 1 letter password, there would be 26 possibilities; one for every letter of the English alphabet. For a 2 letter password, each letter is independent of the other, so there would be 26 times 26 possibilities. Using this information, print the amount of possible passwords that can be formed with 3 letters.
print(26**3)
10. Fill in the blank to calculate how many sectors a given 16 GB (gigabyte) hard disk drive has. The given hard drive is divided into sectors of 512 bytes each. Divide the total bytes on the drive by the number of bytes in a sector to calculate how many sectors this drive has. Your result should be a number. Note: To calculate the total bytes on the disk drive, multiply by multiples of 1024. In the code below, you can calculate the “disk_size” of 16 GB by multiplying 16 by 1024 three times to go from bytes, to kilobytes, to megabytes, and finally to gigabytes.
disk_size = 16*1024*1024*1024
sector_size = 512
sector_amount = ___
print(sector_amount) # Should print 33554432.0
Answer – sector_amount = disk_size / sector_size
Week 2 – Crash Course on Python
Week 2 Quiz – Crash Course on Python
1. Complete the code to output the statement, “Marjery lives at her home address of 1234 Mockingbird Lane”. Remember that precise syntax must be used to receive credit.
name = 'Marjery'
home_address = '1234 Mockingbird Lane'
print(name + " lives at her home address of " + home_address)
2. What’s the value of this Python expression: 7 < “number”?
- True
- False
- TypeError
- 0
3. What directly follows the elif keyword in an elif statement?
- A colon
- A comparison
- A function definition
- A logical operator
4. Consider the following scenario about using if-elif-else statements:
Police patrol a specific stretch of dangerous highway and are very particular about speed limits. The speed limit is 65 miles per hour. Cars going 85 miles per hour or more are given a “Reckless Driving” ticket. Cars going more than 65 miles per hour but less than 85 miles per hour are given a “Speeding” ticket. Any cars going less than 65 are labeled “Safe” in the system.
Fill in the blanks in this function so it returns the proper ticket type or label.
def speeding_ticket(speed):
if speed>=85:
ticket = "Reckless Driving"
elif speed>65:
ticket = "Speeding"
else:
ticket = "Safe"
return ticket
print(speeding_ticket(87)) # Should print Reckless Driving
print(speeding_ticket(66)) # Should print Speeding
print(speeding_ticket(65)) # Should print Safe
print(speeding_ticket(85)) # Should print Reckless Driving
print(speeding_ticket(35)) # Should print Safe
print(speeding_ticket(77)) # Should print Speeding
Question 5
5. What’s the value of the comparison in this if statement? Hint: The answer is not what the code will print.
n = 4
if n*6 > n**2 or n%2 == 0:
print("Check")
- Check
- 24 > 16 or 0
- False
- True
6. Fill in the blanks to complete the function. The “identify_IP” function receives an “IP_address” as a string through the function’s parameters, then it should print a description of the IP address. Currently, the function should only support three IP addresses and return “unknown” for all other IPs.
def identify_IP(IP_address):
if IP_address == "192.168.1.1":
IP_description = "Network router"
elif IP_address == "8.8.8.8" or IP_address == "8.8.4.4":
IP_description = "Google DNS server"
elif IP_address == "142.250.191.46":
IP_description = "Google.com"
else:
IP_description = "unknown"
return IP_description
print(identify_IP("8.8.4.4")) # Should print 'Google DNS server'
print(identify_IP("142.250.191.46")) # Should print 'Google.com'
print(identify_IP("192.168.1.1")) # Should print 'Network router'
print(identify_IP("8.8.8.8")) # Should print 'Google DNS server'
print(identify_IP("10.10.10.10")) # Should print 'unknown'
print(identify_IP("")) # Should Should print 'unknown'
7. Can you calculate the output of this code?
def sum(x, y):
return(x+y)
print(sum(sum(1,2), sum(3,4)))
Answer – 10
8. What’s the value of this Python expression?
x = 5*2
((10 != x) or (10 > x))
- True
- False
- 15
- 10
9. Fill in the blanks to complete the function. The “return_nonnegative” function takes in two numbers and determines which number is larger. Then, it subtracts the smaller number from the larger and returns the result. The result will always be greater than or equal to 0. Complete the body of the function so that it returns the correct result.
def return_nonnegative(first_num, second_num):
if first_num > second_num:
result = first_num - second_num
else:
result = second_num - first_num
return result
print(return_nonnegative(5, 5)) # Should print 0
print(return_nonnegative(4, 5)) # Should print 1
print(return_nonnegative(5, 3)) # Should print 2
print(return_nonnegative(2, 5)) # Should print 3
print(return_nonnegative(5, 0)) # Should print 5
print(return_nonnegative(0, 5)) # Should print 5
10. Code that is written so that it is readable and doesn’t conceal its intent is called what?
- Self-documenting code
- Intentional code
- Obvious code
- Maintainable code
Week 3 – Crash Course on Python
Week 3 Quiz – Crash Course on Python
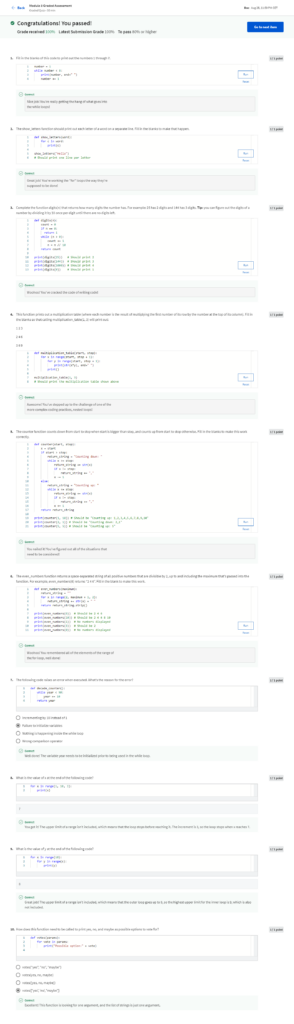
Week 4 – Crash Course on Python
Week 4 Quiz – Crash Course on Python
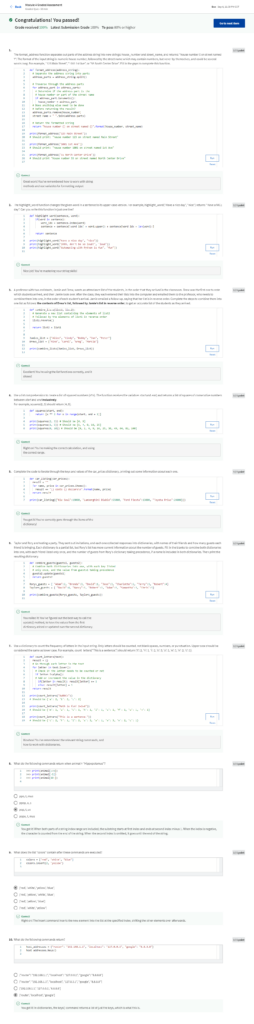
Week 5 – Crash Course on Python
Optional
Week 6 – Crash Course on Python
Week 6 Quiz – Crash Course on Python
1. A programmer is working on a new project and encounters a complex problem. They are tempted to spend a lot of time trying to solve the problem on their own. However, a colleague advises them to research existing solutions first. What is the colleague’s advice based on?
- The colleague believes that the programmer should not waste time reinventing something that already exists.
- The colleague believes that the programmer should not trust their own abilities to solve the problem.
- The colleague believes that the programmer should not try to be innovative.
- The colleague believes that the programmer should ask someone with more experience to solve this problem.
2. Based on the following code, what is the correct output?
numbers = [ 3, 2, 4, 5, 1 ]
numbers.sort()
print(numbers)
- 1, 2, 3, 4, 5
- 3, 1, 5, 4, 2
- 1, 5, 2, 4, 3
- 3, 2, 5, 5, 1
3. Which method is used to sort a list without creating a new list?
- The sort() method
- The sorted() method with a reverse argument.
- The sorted() method
- The sort() method with a reverse argument.
4. You need to sort a list in descending order, which argument should you use in your sorted() method to do this?
- revert = True
- revert = False
- reverse = False
- reverse = True
5. Based on the following code, what is the correct output?
names = [“Carlos”, “Ray”, “Alex”, “Kelly”]
print(sorted(names, key=len))
- [‘Ray’, ‘Alex’, ‘Kelly’, ‘Carlos’]
- [‘Carlos’, ‘Kelly’, ‘Alex’, ‘Ray’]
- [‘Carlos’, ‘Ray’, ‘Alex’, ‘Kelly’]
- [‘Alex’, ‘Ray’, ‘Carlos’, ‘Kelly’]
6. A grocery store is tracking the inventory of its products. For each product, the store needs to keep track of the product name, the product ID, the quantity in stock, and the price. Which data structure is the most efficient for storing the information associated with each product?
- A tuple
- A list
- A dictionary
- A set
7. A software engineer is tasked with developing a system that monitors network traffic and alerts administrators when suspicious activity is detected. The system receives a continuous stream of network traffic data, which needs to be processed and analyzed in real-time. The engineer is unsure of the best way to handle this high-volume data stream efficiently. What should the programmer do?
- Use a third-party library or tool designed for real-time data processing.
- Increase the size of the database server.
- Create 2 separate functions to process the events and print the associated data to the screen.
- Create one function to process the events and print the associated data to the screen.
8. What does the following code snippet do?
if event.type == “login”:
machines[event.machine].add(event.user)
- It removes the user from the list of logged-in users for the specified machine.
- It checks if the event type is “login” and if so, adds the user to the list of logged-in users for a specified machine.
- It checks if the event type is “logout” and if so, removes the user from the list of logged-in users for the specified machine.
- It adds the user to the list of logged-in users for the specified machine.
9. A programmer is designing a grading script for a teacher. The teacher needs to assign grades to students based on different student assessment scores. The programmer needs to create a script that will execute code block A if condition 1 is true but will execute code block B if condition 1 is false and condition 2 is true. What type of statement should the programmer use to evaluate condition 2?
- and statement
- else statement
- if statement
- elif statement
10. Take a look at the code snippet below. What parameters does this method take in? Select all that apply.
class Event:
def __init__(self, event_date, event_type, machine_name, user):
self.date = event_date
self.type = event_type
self.machine = machine_name
self.user = user
- event_type
- event
- machine_name
- event_date
Ypu may like:
How to Become a Full-Stack Web Developer: A Step-by-Step Guide