Angular Components are the building blocks of Angular applications. They allow developers to create reusable and maintainable code, and to build dynamic and interactive user interfaces.
In this article, we will explore the following:
- What are Angular Components?
- Key features of Angular Components
- How to use Angular Components effectively
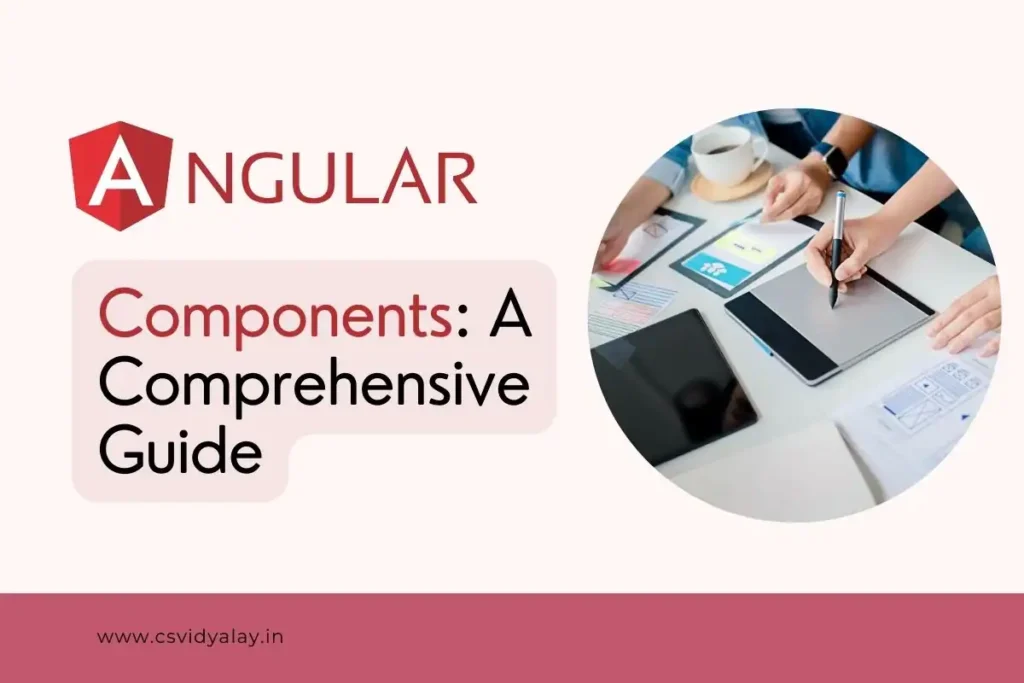
Contents
- 1 What are Angular Components?
- 2 Key Features of Angular Components
- 3 Using Angular Components
- 4 Understanding the Component Lifecycle
- 5 Benefits of using Angular Components
- 6 FAQs
- 6.1 What is the difference between a component and a directive in Angular?
- 6.2 Can a component have multiple templates?
- 6.3 How can I pass data from a parent component to a child component?
- 6.4 How can I communicate from a child component to a parent component?
- 6.5 What are lifecycle hooks in Angular components?
- 6.6 How can I style an Angular component?
- 7 Conclusion
What are Angular Components?
Angular Components are self-contained pieces of UI code that can be reused throughout an application. They encapsulate the HTML, CSS, and TypeScript code required to render a specific part of the user interface.
Angular Components are created using the Angular CLI, which generates a component class, template, and style file. The component class contains the logic and data for the component, while the template defines the UI structure. The style file defines the component’s appearance using CSS.
Must Read:
Top 20 2023 Angular Interview Questions
Key Features of Angular Components
- Templates: Angular components use templates to define the structure and layout of the user interface. Templates are written in HTML and can include Angular-specific syntax and directives to add dynamic behavior.
- Data Binding: Components enable two-way data binding, allowing data to flow seamlessly between the component and its template. This feature ensures that any changes in the component’s data are automatically reflected in the UI, and vice versa.
- Lifecycle Hooks: Angular components provide a set of lifecycle hooks that allow developers to tap into various stages of a component’s lifecycle. These hooks enable actions such as initialization, data retrieval, and cleanup, enhancing the control and flexibility of the application.
- Dependency Injection: Angular’s dependency injection system plays a crucial role in component development. It allows components to declare their dependencies and have them injected automatically, promoting modularity and testability.
Using Angular Components
To create a new component, you can use the Angular CLI command ng generate component component-name
. This command generates the necessary files and folder structure for your component, including the component class, template, and styles.
A typical Angular component consists of three main parts: the component class, the template, and the styles. The component class contains the logic and data for the component, while the template defines the UI structure. The styles define the component’s appearance using CSS or a preprocessor like Sass.
Components can communicate with each other using input and output properties. Input properties allow data to flow into a component, while output properties emit events to notify parent components about specific actions or changes.
Angular components are designed to be reusable. By creating modular and self-contained components, you can easily reuse them across different parts of your application, promoting code maintainability and reducing duplication.
Understanding the Component Lifecycle
The component lifecycle in Angular is the process by which a component is created, initialized, rendered, updated, and destroyed. Angular provides a set of lifecycle hooks that allow developers to tap into these different stages of the component lifecycle and perform specific actions.
Here is a brief overview of the Angular component lifecycle:
- Component creation: When Angular creates a component, it first instantiates the component class. This creates a new instance of the component class, which is then used to render the component’s view.
- Component initialization: Once the component class has been instantiated, Angular calls the
ngOnInit()
lifecycle hook. This hook is used to initialize the component’s state and perform any other necessary setup tasks. - Component rendering: After the
ngOnInit()
hook has been called, Angular renders the component’s view. The view is rendered by compiling the component’s template and applying the component’s styles. - Component update: If any of the component’s input properties change, Angular calls the
ngOnChanges()
lifecycle hook. This hook is used to update the component’s state based on the changes to its input properties. - Component destruction: When a component is destroyed, Angular calls the
ngOnDestroy()
lifecycle hook. This hook is used to clean up any resources that were allocated by the component.
Here are some common use cases for the different Angular component lifecycle hooks:
- ngOnInit(): This hook is typically used to initialize the component’s state and perform any other necessary setup tasks, such as fetching data from a back-end server.
- ngOnChanges(): This hook is typically used to update the component’s state based on the changes to its input properties. For example, if the component has an input property that represents a user’s name, you could use the
ngOnChanges()
hook to update the component’s UI whenever the user’s name changes. - ngOnDestroy(): This hook is typically used to clean up any resources that were allocated by the component, such as unsubscribing from any subscriptions or closing any open file handles.
By understanding the Angular component lifecycle and how to use the different lifecycle hooks, you can write more efficient and maintainable code.
Benefits of using Angular Components
Here are some of the benefits of using Angular components:
- Reusability: Angular components are designed to be reusable. This means that you can create a single component and use it multiple times throughout your application, without having to rewrite the code. This can save you a lot of time and effort, and it can also help to improve the consistency of your application’s UI.
- Maintainability: Angular components are also highly maintainable. This is because they are self-contained and isolated from other components in your application. This makes it easy to make changes to individual components without affecting the rest of your application.
- Encapsulation: Angular components encapsulate the HTML, CSS, and TypeScript code required to render a specific part of the application. This helps to keep your code organized and easy to understand.
- Modularity: Angular components are modular, meaning that they can be combined and composed to create complex user interfaces. This makes it easy to build and maintain large and complex applications.
Must Read:
Angular and AngularJS: Which One Should You Choose in 2023?
FAQs
-
What is the difference between a component and a directive in Angular?
A component is a type of directive with a template. It encapsulates the HTML, CSS, and TypeScript code required to render a specific part of the application. Components are typically used to create reusable UI elements. On the other hand, a directive is a more general concept that allows you to extend the behavior of HTML elements or attributes. Directives can be classified into three types: structural directives, attribute directives, and component directives.
-
Can a component have multiple templates?
No, a component in Angular can have only one template. However, you can use conditional statements and structural directives like ngIf or ngSwitch within the template to control the rendering of different sections based on certain conditions.
-
How can I pass data from a parent component to a child component?
You can pass data from a parent component to a child component using input properties. In the child component, define an input property using the
@Input()
decorator, specifying the name of the property. Then, in the parent component’s template, you can bind the value to the child component’s input property using property binding syntax. -
How can I communicate from a child component to a parent component?
To communicate from a child component to a parent component, you can use output properties and event emitters. In the child component, define an output property using the
@Output()
decorator and an instance of theEventEmitter
class. Emit events from the child component using theemit()
method of the event emitter. In the parent component’s template, bind to the child component’s output property and handle the emitted events using event binding syntax. -
What are lifecycle hooks in Angular components?
Lifecycle hooks are methods that Angular provides at specific stages of a component’s lifecycle. These hooks allow you to perform actions or respond to events during different phases of a component’s existence. Some commonly used lifecycle hooks include
ngOnInit
,ngOnChanges
,ngOnDestroy
, andngAfterViewInit
. You can implement these hooks in your component class to execute custom logic when the corresponding lifecycle event occurs. -
How can I style an Angular component?
You can style an Angular component by using CSS or a preprocessor like Sass. Each component can have its own styles defined in a separate style file, typically with the
.css
extension. You can also use inline styles by specifying thestyles
property in the component’s metadata. Additionally, you can apply global styles to your entire application by adding them to thestyles.css
file in thesrc
folder.
Conclusion
Angular components are the foundation of modern web applications. They offer a structured and modular approach to UI development, enabling developers to create reusable and maintainable code. With Angular components, you can build dynamic and interactive user interfaces that deliver an exceptional user experience.
Whether you’re a seasoned developer or just starting out, Angular components are a must-learn for web development. They offer a powerful and versatile way to create complex user interfaces in a simple and efficient way.
References:
- Angular Documentation: https://angular.io/guide/architecture-components