Ace your Angular interview with these 20+ advanced Angular interview questions for experienced developers. Covering everything from Angular architecture to RxJS, this guide will prepare you for even the most challenging questions.
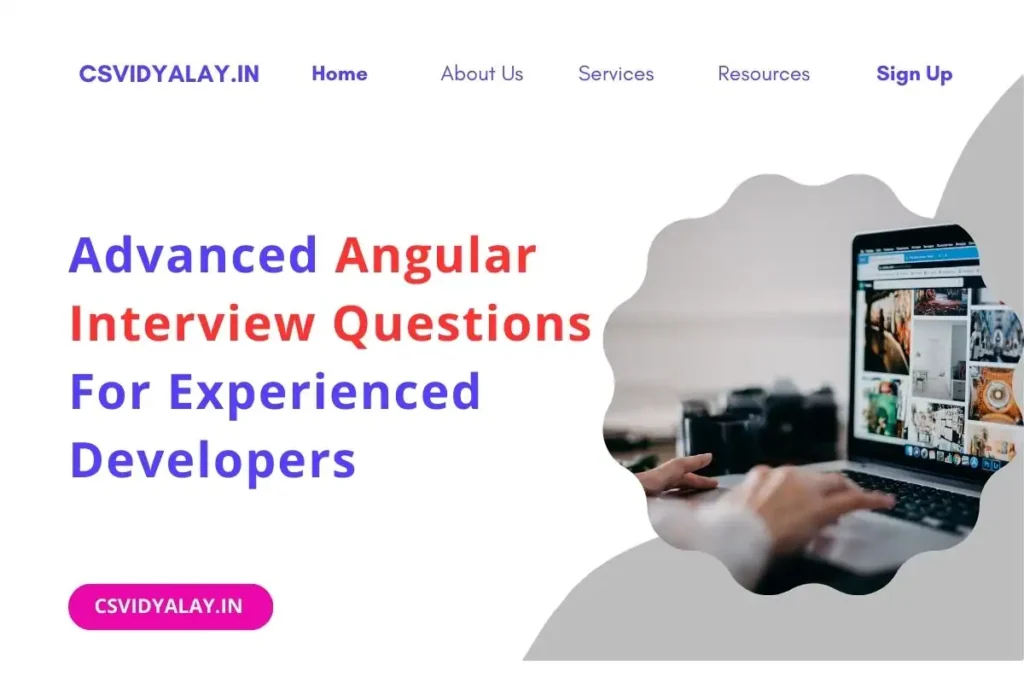
Contents
- 1 Top Angular Interview Questions For Experienced Developers
- 1.1 What are the key features of Angular?
- 1.2 Explain the Angular component lifecycle hooks.
- 1.3 What is the purpose of Angular modules?
- 1.4 How does Angular handle forms and form validation?
- 1.5 What is Angular CLI, and why is it useful?
- 1.6 How does Angular handle HTTP requests?
- 1.7 What is the difference between Angular components and directives?
- 1.8 Explain the concept of lazy loading in Angular.
- 1.9 How does Angular handle dependency injection?
- 1.10 What are Angular services and why are they important?
- 1.11 What is the purpose of Angular decorators and provide some examples.
- 1.12 How can you share data between components in Angular?
- 1.13 What is the role of the Angular router and how does it work?
- 1.14 Explain the concept of Angular pipes and provide some examples.
- 1.15 What is Angular CLI and how can it be used to create a new Angular project?
- 1.16 How can you optimize the performance of an Angular application?
- 1.17 What are Angular guards and how can they be used for route protection?
- 1.18 Explain the concept of Angular animations and provide an example.
- 1.19 How can you handle errors in Angular HTTP requests?
- 1.20 What is the purpose of Angular directives and provide some examples.
- 1.21 How can you implement internationalization (i18n) in an Angular application?
- 1.22 What is the purpose of Angular decorators and provide some examples.
- 1.23 How can you handle form validation in Angular reactive forms?
- 1.24 Explain the concept of Angular change detection and its importance.
- 1.25 What are Angular interceptors and how can they be used?
- 1.26 How can you implement server-side rendering (SSR) in an Angular application?
Top Angular Interview Questions For Experienced Developers
What are the key features of Angular?
Angular offers a number of key features that make it a popular choice for web development, including:
- Two-way data binding: Allows automatic synchronization of data between the model and the view.
- Dependency injection: Helps manage dependencies between different components.
- Directives: Enables the creation of reusable components and custom HTML tags.
- Routing: Facilitates navigation between different views in a single-page application.
- Testing: Provides robust testing capabilities with tools like Jasmine and Karma.
Must Read:
Top 20 2023 Angular Interview Questions
Explain the Angular component lifecycle hooks.
Angular components have several lifecycle hooks that allow developers to perform actions at specific stages of a component’s lifecycle. The main lifecycle hooks are:
- ngOnInit: Called after the component is initialized and its inputs are bound.
- ngOnChanges: Called when the component’s input properties change.
- ngOnDestroy: Called just before the component is destroyed.
- ngAfterViewInit: Called after the component’s view has been initialized.
- ngAfterContentInit: Called after the component’s content has been initialized.
What is the purpose of Angular modules?
Angular modules are used to organize and encapsulate related components, directives, services, and other code. They help in managing the application’s complexity and enable code reusability. Angular modules can be feature modules or root modules, and they define the boundaries of the application’s functionality.
How does Angular handle forms and form validation?
Angular provides two approaches for handling forms: template-driven forms and reactive forms.
- Template-driven forms: This approach relies on directives in the template to create and manage the form. Validation is done using built-in directives or custom validators.
- Reactive forms: This approach uses explicit form control objects in the component class to manage the form. It provides more flexibility and control over form validation.
What is Angular CLI, and why is it useful?
Angular CLI (Command Line Interface) is a powerful tool that helps developers scaffold, build, and maintain Angular applications. It provides a set of commands to generate components, services, modules, and more. Angular CLI also offers features like code scaffolding, automatic code formatting, and easy project configuration, making it an essential tool for Angular development.
How does Angular handle HTTP requests?
Angular provides the HttpClient module to handle HTTP requests. It offers a simplified API for making GET, POST, PUT, DELETE, and other HTTP requests. The HttpClient module also supports interceptors, which allow developers to modify requests or responses globally.
What is the difference between Angular components and directives?
Feature | Angular component | Angular directive |
---|---|---|
Definition | A building block of an Angular application that defines the structure, behavior, and appearance of a part of the user interface. | An instruction in the DOM that tells Angular how to manipulate DOM elements. |
Template | Has its own template, styles, and logic. | Can or cannot have its own template. |
Types | None | Structural, attribute, and component directives. |
Explain the concept of lazy loading in Angular.
Lazy loading is a technique in Angular that allows you to load modules and their associated components only when they are needed. This helps in reducing the initial bundle size and improves the application’s performance.
To implement lazy loading, you need to split your application into multiple feature modules. Each feature module can be loaded separately when the user navigates to a specific route that requires that module.
How does Angular handle dependency injection?
Dependency injection (DI) is a design pattern used in Angular to provide dependencies to a class or component. Angular’s DI system allows you to define dependencies in the constructor of a class or component, and Angular takes care of creating and providing the required instances.
Angular’s DI system uses a hierarchical injector tree to resolve dependencies. When a component requests a dependency, Angular looks for the dependency in its own injector. If it’s not found, Angular continues to search for the dependency in the parent injectors until it reaches the root injector.
Must Read:
Angular and AngularJS: Which One Should You Choose in 2023?
What are Angular services and why are they important?
Angular services are classes that are responsible for providing specific functionality and data to different parts of an application. They are used to share data, perform business logic, and interact with external APIs or databases.
Services play a crucial role in promoting code reusability, separation of concerns, and maintainability. They help in keeping the components lean and focused on their specific tasks, while the services handle the complex logic and data management.
What is the purpose of Angular decorators and provide some examples.
Angular decorators are functions that modify the behavior of classes, methods, or properties in Angular. They are used to add metadata to classes, which Angular uses to understand and process the code.
Some examples of Angular decorators are:
- @Component: Used to define an Angular component.
- @Directive: Used to define an Angular directive.
- @Injectable: Used to define an Angular service.
- @Input: Used to define an input property in a component or directive.
- @Output: Used to define an output property in a component or directive.
Decorators provide a way to extend and customize the behavior of Angular elements, making them a powerful tool for building Angular applications.
There are several ways to share data between components in Angular:
- Using @Input and @Output: Parent components can pass data to child components using @Input properties, and child components can emit events using @Output properties to communicate with the parent component.
- Using a shared service: Components can share data through a shared service by injecting the service into both components. The service can store and provide data that needs to be shared between components.
- Using a state management library: Libraries like NgRx or Akita can be used to manage application state and share data between components using a centralized store.
The choice of method depends on the complexity and requirements of the application.
What is the role of the Angular router and how does it work?
The Angular router is responsible for managing navigation and routing in an Angular application. It allows users to navigate between different views or pages without reloading the entire application.
The router works by mapping URLs to specific components and rendering the corresponding view. It uses a configuration file where you define the routes and their associated components. When a user navigates to a specific URL, the router matches the URL to a route and renders the corresponding component.
The router also provides features like route parameters, route guards, lazy loading, and child routes, making it a powerful tool for building complex navigation structures in Angular applications.
Explain the concept of Angular pipes and provide some examples.
Angular pipes are a feature that allows you to transform and format data in templates. They are used to modify the appearance or behavior of data before displaying it to the user.
Some examples of Angular pipes are:
- DatePipe: Used to format dates.
- CurrencyPipe: Used to format currency values.
- UpperCasePipe: Used to convert text to uppercase.
- LowerCasePipe: Used to convert text to lowercase.
- DecimalPipe: Used to format decimal numbers.
Pipes can be chained together to perform multiple transformations on data. Custom pipes can also be created to meet specific formatting or transformation requirements.
What is Angular CLI and how can it be used to create a new Angular project?
- Angular CLI (Command Line Interface) is a powerful tool that helps developers scaffold, build, and maintain Angular applications. It provides a set of commands to generate components, services, modules, and more.
To create a new Angular project using Angular CLI, you can run the following command in your terminal:
ng new project-name
This command creates a new Angular project with the specified name. Angular CLI will generate the project structure, install the necessary dependencies, and set up a basic configuration.
How can you optimize the performance of an Angular application?
- Minimize the bundle size:
- Lazy load modules: Load modules and their associated components only when they are needed.
- Use code splitting techniques: Split your application into multiple bundles, and load them only when needed.
- Use AOT compilation:
- Reduce the size of the generated JavaScript code by compiling the code ahead of time.
- Optimize the loading time:
- Leverage browser caching and compression techniques to reduce the number of requests and the size of the downloaded resources.
- Use Angular’s change detection strategy wisely:
- Use the OnPush change detection strategy to avoid unnecessary re-rendering of components.
- Implement server-side rendering (SSR):
- Improve the initial load time and SEO by rendering the application on the server and sending the pre-rendered HTML to the client.
- Optimize the usage of third-party libraries:
- Avoid unnecessary dependencies and use third-party libraries wisely.
- Use Angular’s production build mode:
- Enable additional optimizations like tree shaking and dead code elimination in the production build.
What are Angular guards and how can they be used for route protection?
Angular guards are used to protect routes in an Angular application. They allow you to control access to certain routes based on specific conditions or user roles.
There are different types of guards in Angular:
- CanActivate: Determines if a user can access a route.
- CanActivateChild: Determines if a user can access the child routes of a route.
- CanDeactivate: Determines if a user can leave a route.
- CanLoad: Determines if a user can load a lazy-loaded module.
You can implement custom guard logic to check authentication, authorization, or any other conditions before allowing access to a route. Guards can be applied to individual routes or to a group of routes.
Here are some examples of how to use Angular guards:
// Protect a route for authenticated users only:
import { CanActivate } from '@angular/router';
@Injectable({ providedIn: 'root' })
export class AuthGuard implements CanActivate {
constructor(private authService: AuthService) {}
canActivate(): boolean {
return this.authService.isAuthenticated();
}
}
// Apply the guard to a route:
{
path: 'admin',
component: AdminComponent,
canActivate: [AuthGuard]
}
// Protect a group of routes for authenticated users only:
import { CanActivateChild } from '@angular/router';
@Injectable({ providedIn: 'root' })
export class AdminGuard implements CanActivateChild {
constructor(private authService: AuthService) {}
canActivateChild(): boolean {
return this.authService.isAuthenticated();
}
}
// Apply the guard to a group of routes:
{
path: 'admin',
children: [
{
path: 'users',
component: UsersComponent,
canActivateChild: [AdminGuard]
},
{
path: 'products',
component: ProductsComponent,
canActivateChild: [AdminGuard]
}
]
}
Explain the concept of Angular animations and provide an example.
- Angular animations allow you to add rich and interactive animations to your Angular applications. They can be used to create smooth transitions, visual effects, and user interactions.
To use animations in Angular, you need to define animation states, transitions, and triggers. Animation states represent different states of an element, transitions define how the element moves between states, and triggers are used to activate the animations.
Here’s an example of a fade-in animation in Angular:
import { trigger, state, style, animate, transition } from '@angular/animations';
@Component({
selector: 'app-example',
templateUrl: './example.component.html',
styleUrls: ['./example.component.css'],
animations: [
trigger('fadeInOut', [
state('void', style({ opacity: 0 })),
transition(':enter, :leave', [
animate(500, style({ opacity: 1 }))
])
])
]
})
export class ExampleComponent {
isVisible = false;
toggleVisibility() {
this.isVisible = !this.isVisible;
}
}
In the above example, the fadeInOut animation is triggered when the component is added or removed from the DOM. The animation gradually changes the opacity of the element over a duration of 500 milliseconds.
How can you handle errors in Angular HTTP requests?
- Handling errors in Angular HTTP requests: To handle errors in Angular HTTP requests, you can use the catchError operator from the RxJS library. This operator allows you to catch and handle errors emitted by an observable.
Here’s an example of handling errors in an HTTP request:
import { HttpClient, HttpErrorResponse } from '@angular/common/http';
import { catchError } from 'rxjs/operators';
import { throwError } from 'rxjs';
@Injectable()
export class DataService {
constructor(private http: HttpClient) {}
getData() {
return this.http.get('api/data').pipe(
catchError((error: HttpErrorResponse) => {
// Handle the error here
console.error('An error occurred:', error.message);
return throwError('Something went wrong. Please try again later.');
})
);
}
}
In the above example, the catchError operator is used to catch any errors that occur during the HTTP request. The error is then logged to the console, and a custom error message is returned using the throwError function.
What is the purpose of Angular directives and provide some examples.
Angular directives are instructions in the DOM that tell Angular how to manipulate the DOM elements. They allow you to extend HTML with custom behavior and create reusable components.
Here are some examples of Angular directives:
- ngIf: Conditionally adds or removes an element from the DOM based on a condition.
- ngFor: Renders a template for each item in a collection.
- ngStyle: Dynamically applies CSS styles to an element.
- ngClass: Dynamically adds or removes CSS classes from an element.
- ngModel: Binds an input, select, or textarea element to a property in the component.
Directives provide a way to enhance the functionality and behavior of HTML elements in Angular applications.
How can you implement internationalization (i18n) in an Angular application?
Angular provides built-in support for internationalization (i18n) to create applications that can be easily translated into different languages.
To implement i18n in an Angular application, you can follow these steps:
- Mark translatable text in templates using the
i18n
attribute. - Extract the translatable text using the Angular CLI command:
ng xi18n
. - Translate the extracted text in the generated translation files.
- Configure the application to use the translated files by providing the appropriate locale.
- Build the application with the translated files using the Angular CLI command:
ng build --prod --localize
.
By following these steps, you can create an Angular application that supports multiple languages and can be easily translated.
Here are some additional tips for implementing i18n in your Angular applications:
- Use the
i18n
attribute to mark all translatable text in your templates. This will make it easier to extract the translatable text and translate it into different languages. - Use the
ng xi18n
command to extract the translatable text and generate the translation files. - Translate the extracted text in the generated translation files. Be sure to use the appropriate locale for each translation file.
- Configure the application to use the translated files by providing the appropriate locale. You can do this in the
angular.json
file. - Build the application with the translated files using the
ng build --prod --localize
command. This will generate a production-ready build of your application with the translated files embedded.
By following these tips, you can create Angular applications that are ready for global audiences.
Must Read:
Angular Components: What They Are and How to Use Them
What is the purpose of Angular decorators and provide some examples.
Angular decorators are functions that modify the behavior of classes, methods, or properties in Angular applications. They provide metadata that Angular uses to enhance the functionality of the application.
Some examples of Angular decorators are:
- @Component: Used to decorate a class as an Angular component, providing metadata such as the selector, template, styles, and more.
- @Injectable: Used to decorate a class as an injectable service, allowing it to be injected into other components or services.
- @Input: Used to decorate a property in a component, making it bindable from its parent component.
- @Output: Used to decorate an event emitter property in a component, allowing it to emit events to its parent component.
- @Directive: Used to decorate a class as an Angular directive, providing metadata for custom behavior on DOM elements.
Angular decorators play a crucial role in configuring and extending the functionality of Angular components, services, and directives.
How can you handle form validation in Angular reactive forms?
Angular provides a powerful form module that allows you to handle form validation in a reactive manner. Here’s how you can handle form validation in Angular reactive forms:
- Import the necessary form-related classes from
@angular/forms
module. - Create an instance of
FormGroup
to represent the form and its controls. - Define form controls using
FormControl
orFormGroup
instances. - Apply validation rules to form controls using built-in validators or custom validators.
- Bind form controls to HTML input elements using the
formControlName
directive. - Display validation errors in the template using Angular’s
ngIf
directive and theerrors
property of form controls. - Handle form submission and perform additional validation logic in the component.
By following these steps, you can implement robust form validation in Angular reactive forms, ensuring that user input meets the desired criteria.
Explain the concept of Angular change detection and its importance.
Angular change detection is the process by which Angular determines if there are any changes in the application’s data and updates the view accordingly. It is a critical mechanism that ensures the synchronization between the model and the view.
Angular uses a hierarchical tree structure to perform change detection. When an event occurs or data changes, Angular starts the change detection process from the root component and traverses down the component tree. It checks each component’s properties and bindings to detect any changes and updates the view accordingly.
The importance of Angular change detection lies in its ability to provide a seamless and reactive user experience. By automatically updating the view when data changes, Angular ensures that the user interface is always up to date and reflects the current state of the application.
What are Angular interceptors and how can they be used?
Angular interceptors are a powerful feature that allows you to intercept and modify HTTP requests and responses. They provide a way to add custom logic, such as authentication, error handling, or request/response manipulation, to the HTTP pipeline.
To use Angular interceptors, you need to create a class that implements the HttpInterceptor
interface. This class can then be registered as a provider in the Angular module.
Interceptors can be used for various purposes, such as:
- Adding authentication headers to outgoing requests.
- Handling errors and displaying appropriate error messages.
- Modifying request/response data.
- Logging HTTP requests and responses.
- Caching responses to improve performance.
By using interceptors, you can centralize and reuse common HTTP-related logic across your Angular application, making it easier to maintain and enhance.
How can you implement server-side rendering (SSR) in an Angular application?
Server-side rendering (SSR) is a technique that allows you to render Angular applications on the server and send the pre-rendered HTML to the client. This improves the initial load time and enables search engines to crawl and index the application.
To implement SSR in an Angular application, you need to follow these steps:
- Set up a server-side rendering platform, such as Angular Universal.
- Create server-side versions of your Angular components.
- Configure the server to handle incoming requests and render the Angular application on the server.
- Set up routing and handle data fetching on the server.
- Build and deploy the server-side rendered application.
By implementing SSR, you can improve the performance and SEO-friendliness of your Angular application.