Are you preparing for a React interview? Here are the top 20 React hook interview questions to help you ace it! This blog post covers everything from the most basic React hook questions to more advanced ones, so there’s something for everyone.
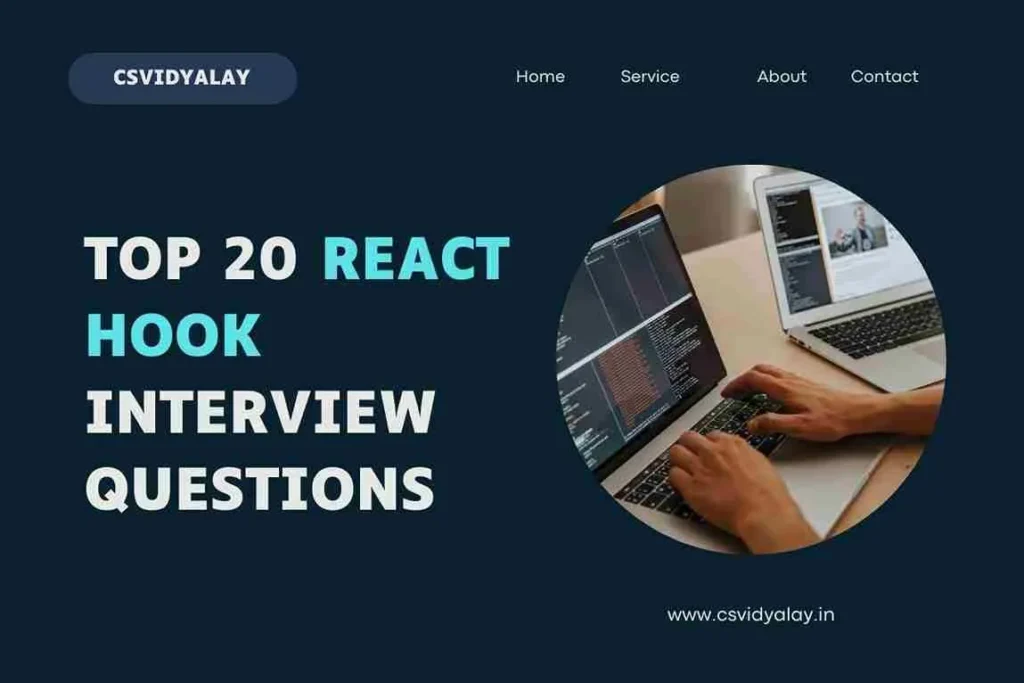
Contents
- 1 Top React Hook Interview Questions
- 1.1 1. What are React Hooks?
- 1.2 2. What are the benefits of using React Hooks?
- 1.3 3. How do you create a custom React Hook?
- 1.4 4. What is the difference between useState and useEffect Hooks?
- 1.5 5. How do you handle side effects in React Hooks?
- 1.6 6. What is the purpose of the useContext Hook?
- 1.7 7. How do you optimize performance in React Hooks?
- 1.8 8. What is the useReducer Hook and when would you use it?
- 1.9 9. How do you handle form input with React Hooks?
- 1.10 10. What is the useRef Hook used for?”
- 1.11 11. How do you test React Hooks?
- 1.12 12. What are the rules of Hooks in React?
- 1.13 13. How do you handle async operations with React Hooks?
- 1.14 14. What is the useCallback Hook and when would you use it?
- 1.15 15. How do you handle component lifecycle methods with React Hooks?
- 1.16 16. What is the useMemo Hook and when would you use it?
- 1.17 17. How do you handle context in React Hooks?
- 1.18 18. What is the useLayoutEffect Hook and how is it different from useEffect?
- 1.19 19. How do you handle error boundaries with React Hooks?
- 1.20 20. What are the best practices for using React Hooks?
Top React Hook Interview Questions
Must Read:
How to Become a Full-Stack Web Developer: A Step-by-Step Guide
1. What are React Hooks?
React Hooks are functions that let you use state and other React features in functional components. They were introduced in React 16.8 to make it easier to write reusable code and manage states without using class components.
With Hooks, you can:
- Encapsulate and reuse stateful logic across components
- Write simpler and more concise code
- Improve the readability and maintainability of your code
- Get better performance and easier testing
If you’re writing React code, you should definitely be using Hooks. They’re a powerful tool for building more efficient and scalable applications.
2. What are the benefits of using React Hooks?
Here are some of the benefits of using React Hooks:
- Reusability: Hooks make it easy to encapsulate and reuse stateful logic across components. This can help you to write more modular and maintainable code.
- Simplicity: Hooks simplify the code by removing the need for class components and lifecycle methods. This makes it easier to understand and write React code.
- Readability: Hooks promote a more linear and readable code structure. This makes it easier to reason about the component’s behavior and to identify and fix bugs.
- Performance: Hooks can improve performance by optimizing the way React manages state and re-renders components.
- Testability: Hooks make it easier to write unit tests for components. This is because they separate the concerns of stateful logic from the UI.
3. How do you create a custom React Hook?
To create a custom React Hook, you need to follow a few conventions:
- Name the hook with the prefix “use” to indicate that it’s a custom hook.
- Define the hook as a regular JavaScript function.
- Use built-in hooks or other custom hooks within your custom hook.
- Return the necessary values or functions that other components can use.
For example, let’s say you want to create a custom hook to handle a counter:
import { useState } from 'react';
function useCounter(initialValue) {
const [count, setCount] = useState(initialValue);
const increment = () => {
setCount(count + 1);
};
return [count, increment];
}
In this example, the custom hook useCounter uses the useState hook internally to manage the count state and returns the current count value and an increment function.
4. What is the difference between useState and useEffect Hooks?
useState and useEffect are two of the most important React Hooks, used to manage state and side effects, respectively.
useState allows you to create and update state variables in functional components, similar to how this.state
works in class components. It takes an initial value as an argument and returns an array with two elements: the current state value and a function to update that value.
useEffect allows you to perform side effects in functional components, such as fetching data, subscribing to events, or updating the DOM. It takes a callback function as an argument, which is executed after the component renders. You can also pass an optional array of dependencies to useEffect
, which determines when the effect should be re-run.
In summary:
- useState is used for managing state within a component.
- useEffect is used for handling side effects like data fetching, subscriptions, or DOM updates.
5. How do you handle side effects in React Hooks?
To handle side effects in React Hooks, you can use the useEffect Hook. The useEffect Hook allows you to perform side effects after the component has been rendered.
Here’s an example of how to use useEffect to fetch data from an API when the component mounts:
import React, { useState, useEffect } from 'react';
function MyComponent() {
const [data, setData] = useState(null);
useEffect(() => {
const fetchData = async () => {
const response = await fetch('https://api.example.com/data');
const result = await response.json();
setData(result);
};
fetchData();
}, []);
return (
<div>
{data ? (
<ul>
{data.map((item) => (
<li key={item.id}>{item.name}</li>
))}
</ul>
) : (
<p>Loading...</p>
)}
</div>
);
}
In this example, the useEffect Hook is used to fetch data from an API when the component mounts. The empty dependency array [] ensures that the effect runs only once. The fetched data is stored in the data state variable, and the component renders the data once it’s available.
6. What is the purpose of the useContext Hook?
The useContext Hook in React allows components to access context values without the need to pass them down as props. This can make it easier to share state or data across different components in your application, especially if those components are deeply nested.
In other words, useContext allows you to avoid “prop drilling,” which is when you have to pass props down through a chain of nested components. This can make your code more complex and difficult to maintain.
For example, imagine you have a global theme variable that you want to access from multiple components in your application. Without useContext, you would have to pass the theme prop down through each component until it reached the components that needed it. This could be tedious and error-prone, especially if you have a lot of nested components.
With useContext, you can simply create a context object and then use the useContext Hook to access the context value from any component in your application. This makes it much easier to share global state or data without prop drilling.
Here is an example of how to use useContext:
// Create a context object
const ThemeContext = createContext("light");
// Provide the context value to all child components
function App() {
return (
<ThemeContext.Provider value="dark">
<ChildComponent />
</ThemeContext.Provider>
);
}
// Access the context value from the child component
function ChildComponent() {
const theme = useContext(ThemeContext);
return (
<div style={{ backgroundColor: theme }}>
This component is using the dark theme.
</div>
);
}
In this example, the ThemeContext object is created and the context value is set to “dark.” The ThemeContext.Provider component is then used to provide the context value to all child components.
The ChildComponent component can then access the context value using the useContext Hook. This allows the ChildComponent component to use the dark theme without having to receive the theme prop as a prop.
7. How do you optimize performance in React Hooks?
- Use
useMemo()
to memoize expensive computations and avoid unnecessary re-renders. This is helpful for computationally intensive operations or calculations that don’t need to be re-evaluated on every render. - Use
useCallback()
to memoize event handlers and prevent unnecessary re-creation of functions. This is useful when passing callbacks to child components, as it ensures that the callback reference remains the same unless the dependencies change. - Use
React.memo()
to memoize functional components and prevent re-rendering when props haven’t changed. This is beneficial for optimizing the rendering of components that receive the same props frequently.
8. What is the useReducer Hook and when would you use it?
useReducer is a React hook that allows you to manage complex state in a more organized and predictable way. It is an alternative to useState, and it is particularly useful when dealing with states that involve multiple sub-values or when the next state depends on the previous state.
To use useReducer, you provide it with a reducer function and an initial state. The reducer function takes the current state and an action object as input, and it returns the new state. Actions are objects that describe how you want the state to change.
For example, here is a simple reducer function that increments a counter:
const counterReducer = (state, action) => {
switch (action.type) {
case 'INCREMENT':
return state + 1;
default:
return state;
}
};
To use this reducer function, you would first initialize the state with a value of 0:
const [count, dispatch] = useReducer(counterReducer, 0);
Then, you could dispatch actions to the reducer to increment the counter:
dispatch({ type: 'INCREMENT' });
The reducer function would then return a new state with the counter incremented by 1.
useReducer can be used to manage much more complex states as well. For example, you could use it to manage the state of a shopping cart, a to-do list, or a form.
Must Read:
Angular and AngularJS: Which One Should You Choose in 2023?
9. How do you handle form input with React Hooks?
React Hooks allows you to manage form input easily. To do this, you can use the useState Hook to create a state variable for each form input field. Then, you can update the state whenever the input value changes.
To capture the user’s input and update the corresponding state variable, you can use the onChange event of the input field. This way, you can easily access and manage the form input values within your component.
Here is a simple example:
import React, { useState } from 'react';
const Form = () => {
const [name, setName] = useState('');
const handleChange = (event) => {
setName(event.target.value);
};
return (
<form>
<input
type="text"
name="name"
value={name}
onChange={handleChange}
/>
<button type="submit">Submit</button>
</form>
);
};
export default Form;
In this example, we create a state variable called name
to store the user’s input. We then use the onChange event of the input field to update the name
state variable whenever the user changes the input value.
We can then access the user’s input value by simply reading the name
state variable. For example, we could display the user’s input value in a heading like this:
<h1 className="name">{name}</h1>
Using React Hooks to handle form input is a simple and effective way to manage your form state.
10. What is the useRef Hook used for?”
The useRef Hook in React lets you create a mutable reference that persists across renders. This means that the value of the ref can be changed without causing a re-render. useRef can be used to access DOM elements, store mutable values, or interact with third-party libraries that require direct access to DOM elements.
Here are some examples of how to use useRef:
- Get a reference to an input field and access its value or focus it programmatically.
- Store the previous value of a state variable to compare it to the current value.
- Interact with a third-party library that requires direct access to a DOM element, such as a Canvas or WebGL library.
Here is a simple example of how to use useRef to access an input field and focus it programmatically:
import React, { useRef } from "react";
const Input = () => {
const inputRef = useRef();
const focusInput = () => {
inputRef.current.focus();
};
return (
<input
ref={inputRef}
type="text"
placeholder="Enter your name"
/>
);
};
To focus the input field, you can simply call the focusInput()
function. This will cause the useRef to focus the input element.
useRef is a powerful tool that can be used for a variety of purposes in React. If you find yourself needing to access a DOM element or store a mutable value that persists between renders, useRef is a good option to consider.
11. How do you test React Hooks?
To test React Hooks effectively using Jest and Enzyme:
- Set up your testing environment: Install Jest and Enzyme, and configure them to work with your React project. You may also need to install additional testing libraries like react-test-renderer.
- Import the necessary dependencies: In your test file, import the React component that uses the Hooks you want to test, as well as any other dependencies required for testing.
- Render the component: Use Enzyme’s shallow or mount function to render the component that uses the Hooks. This allows you to access and interact with the rendered output.
- Simulate events and interactions: Use Enzyme’s API to simulate user interactions or trigger events that would trigger the Hooks you want to test. For example, you can simulate a button click or input change.
- Assert the expected behavior: After simulating the necessary events, use Jest’s expect function to assert the expected behavior of the Hooks.
Here is an example of a test for a React component that uses the useState Hook:
import React from "react";
import { shallow } from "enzyme";
import MyComponent from "./MyComponent";
const useStateSpy = jest.spyOn(React, "useState");
useStateSpy.mockImplementation((initialState) => [initialState, jest.fn()]);
const wrapper = shallow(<MyComponent />);
it("should update state on input change", () => {
const input = wrapper.find("input");
input.simulate("change", { target: { value: "new value" } });
expect(useStateSpy).toHaveBeenCalledWith("initial value");
expect(useStateSpy.mock.calls[0][1]).toHaveBeenCalledWith("new value");
});
12. What are the rules of Hooks in React?
- Call Hooks at the top level: Hooks should only be called at the top level of a function component or another custom hook. They should not be called inside loops, conditions, or nested functions. This ensures that Hooks are called consistently and in the same order on every render.
- Call Hooks in the same order: Hooks should always be called in the same order within a component. React relies on the order of Hooks to associate the stateful values with the corresponding hooks. Calling Hooks in a different order can lead to bugs and unexpected behavior.
- Call Hooks from React components: Hooks should only be called from React function components or custom hooks. They should not be called from regular JavaScript functions, event handlers, or asynchronous functions. This ensures that Hooks are used within the React component lifecycle and can access the necessary React state and context.
- Specify consistent dependencies: When using Hooks like useEffect or useCallback, it’s important to specify the dependencies correctly. The dependencies determine when the effect or memoized callback function should be re-evaluated. Missing or incorrect dependencies can lead to stale data or unnecessary re-renders.
By following these rules, you can ensure that Hooks are used correctly and consistently in your React components. Adhering to these rules helps maintain the integrity and reliability of your codebase, making it easier to understand, test, and maintain.
13. How do you handle async operations with React Hooks?
To handle async operations with React Hooks, you can use the useEffect hook in combination with async/await or Promises. Here’s a step-by-step guide on how to handle async operations with React Hooks:
- Import the necessary dependencies: In your functional component, import the useEffect hook from the ‘react’ package.
- Define an async function: Create an async function that encapsulates your async operation. This function can be defined within your component or imported from another module.
- Use the useEffect hook: Within your component, use the useEffect hook to perform the async operation. Pass the async function as the first argument to useEffect.
- Handle the async operation: Inside the useEffect callback, call the async function and handle the returned Promise. You can use either async/await or Promises to handle the async operation.
- Using async/await: If your async function returns a Promise, you can use the await keyword to wait for the Promise to resolve. For example:
useEffect(() => {
const fetchData = async () => {
try {
const data = await fetchSomeData();
// Handle the data
} catch (error) {
// Handle the error
}
};
fetchData();
}, []);
Using Promises: If your async function returns a Promise, you can use the then() and catch() methods to handle the resolved value or any errors. For example:
useEffect(() => {
const fetchData = () => {
fetchSomeData()
.then((data) => {
// Handle the data
})
.catch((error) => {
// Handle the error
});
};
fetchData();
}, []);
Specify dependencies: If your async operation depends on any variables or props, make sure to include them as dependencies in the second argument of useEffect. This ensures that the effect is re-triggered whenever the dependencies change.
14. What is the useCallback Hook and when would you use it?
useCallback is a React hook that memoizes functions. It returns a memoized version of a callback function that only changes if one of its dependencies changes. This can improve performance by avoiding unnecessary re-renders caused by passing new function references to child components.
Here’s an example of how to use the useCallback hook:
import React, { useCallback } from 'react';
function MyComponent() {
const handleClick = useCallback(() => {
// Handle the click event
}, []);
return (
<button onClick={handleClick}>Click me</button>
);
}
In the example above, the useCallback hook is used to memoize the handleClick function. The empty dependency array [] indicates that there are no dependencies, so the memoized function will always be the same. This ensures that the handleClick function reference remains stable across renders, preventing unnecessary re-renders of child components that receive this function as a prop.
Use the useCallback hook when:
- Passing callbacks to child components: If a parent component renders child components and passes down callbacks as props, useCallback can prevent unnecessary re-renders of the child components when the parent component re-renders. This is especially useful for deeply nested components or components that render frequently.
- Optimizing performance: If a callback function is computationally expensive or relies on heavy calculations, useCallback can help optimize performance by memoizing the function and preventing unnecessary recalculations.
- Preventing infinite loops: In certain scenarios, useCallback with dependencies can help prevent infinite loops caused by re-rendering. By specifying the dependencies correctly, you can ensure that the memoized function is updated only when necessary.
15. How do you handle component lifecycle methods with React Hooks?
With React Hooks, you can handle component lifecycle methods using the useEffect
hook. This allows you to replicate the behavior of componentDidMount
, componentDidUpdate
, and componentWillUnmount
in a more declarative and concise manner.
To handle component lifecycle methods with React Hooks:
- Import the
useEffect
hook from thereact
package. - Within your component, use the
useEffect
hook to handle the component lifecycle. Pass a callback function as the first argument touseEffect
. - In the second argument of
useEffect
, specify the dependencies that the effect depends on. This determines when the effect should be triggered and re-evaluated. If the dependencies array is empty, the effect will only run once, similar tocomponentDidMount
. - Inside the
useEffect
callback, you can handle the logic that corresponds to different lifecycle stages:- componentDidMount: To replicate the behavior of
componentDidMount
, you can perform any initial setup or data fetching inside theuseEffect
callback. - componentDidUpdate: To replicate the behavior of
componentDidUpdate
, you can include the necessary dependencies in the second argument ofuseEffect
. When any of the dependencies change, the effect will be triggered, allowing you to handle the necessary updates or side effects. - componentWillUnmount: To replicate the behavior of
componentWillUnmount
, you can return a cleanup function from theuseEffect
callback. This cleanup function will be executed when the component is unmounted, allowing you to clean up any resources or subscriptions.
- componentDidMount: To replicate the behavior of
Example:
import React, { useEffect } from 'react';
function MyComponent() {
useEffect(() => {
// componentDidMount logic
// Perform initial setup or data fetching here
return () => {
// componentWillUnmount logic
// Clean up any resources or subscriptions here
};
}, [/* dependencies */]);
// Render your component here
return <div>My Component</div>;
}
By using the useEffect hook and specifying the appropriate dependencies, you can handle the component lifecycle logic in a more concise and readable manner compared to traditional class-based components.
Benefits of using the useEffect
hook:
- More declarative and concise: The
useEffect
hook allows you to handle component lifecycle methods in a more declarative and concise manner. This makes your code more readable and maintainable. - Fewer side effects: The
useEffect
hook helps you to avoid side effects in your code by encapsulating them within the callback function. This makes your code more predictable and less error-prone. - Better performance: The
useEffect
hook can improve the performance of your application by avoiding unnecessary re-renders. This is because theuseEffect
hook will only trigger the effect when the dependencies change.
Overall, the useEffect
hook is a powerful tool that can help you to write better React components.
16. What is the useMemo Hook and when would you use it?
The useMemo hook in React caches the result of a function or computation, so you don’t have to recalculate it every time the component renders. This can improve performance, especially if the function or computation is expensive.
To use useMemo, you pass it a function and an array of dependencies. The function is called only when one of the dependencies changes. The return value of the function is cached and returned by useMemo.
Here’s an example of how to use the useMemo hook:
import React, { useMemo } from 'react';
function MyComponent({ data }) {
const processedData = useMemo(() => {
// Perform expensive computations or calculations here
// Return the result that will be memoized
return processData(data);
}, [data]);
return (
<div>{processedData}</div>
);
}
In the example above, the useMemo hook is used to memoize the result of the processData function. The function is only recomputed when the ‘data’ dependency changes. This ensures that the expensive computations are performed only when necessary, optimizing the performance of the component.
When to use the useMemo hook:
- Expensive computations: If a component performs expensive computations that don’t need to be recomputed on every render, you can use useMemo to memoize the result. This can improve performance by avoiding unnecessary recalculations.
- Infrequent changes: If a component depends on a value that changes infrequently, but the component itself re-renders frequently, you can use useMemo to memoize the value and prevent unnecessary re-renders. This is useful for dealing with large datasets or complex data transformations.
- Performance optimization: By memoizing the result of a function or computation, you can optimize the performance of your application by reducing the amount of work done during each render cycle. This can lead to faster rendering and a better user experience.
17. How do you handle context in React Hooks?
In React Hooks, you can handle context using the useContext hook. The useContext hook allows you to access the value of a context directly within a functional component, without the need for a Context.Consumer component. Here’s how you can handle context in React Hooks:
- Create a context: First, create a context using the createContext function from the ‘react’ package. This will create a context object that holds the value you want to share across components.
import React from 'react';
const MyContext = React.createContext();
- Provide the context value: Wrap the parent component or a higher-level component tree with a Context.Provider component. Pass the value you want to share as a prop to the Provider.
function App() {
const contextValue = 'Hello, Context!';
return (
<MyContext.Provider value={contextValue}>
{/* Render your components here */}
</MyContext.Provider>
);
}
- Consume the context value: Inside any child component that needs access to the context value, use the useContext hook to retrieve the value.
import React, { useContext } from 'react';
function MyComponent() {
const contextValue = useContext(MyContext);
return (
<div>{contextValue}</div>
);
}
By calling useContext(MyContext), you can access the value provided by the Context.Provider component.
It’s important to note that the useContext hook can only be used within functional components or custom hooks. If you need to access context in a class component, you can still use the Context.Consumer component.
Additionally, make sure that the context you’re accessing with useContext is within the component tree that includes the Context.Provider. If the context is not found, the useContext hook will return the defaultValue provided when creating the context.
18. What is the useLayoutEffect Hook and how is it different from useEffect?
The main difference between useLayoutEffect and useEffect is when they are executed:
- useEffect: Runs asynchronously after the component has rendered and the browser has painted the screen. This means that the user might see the content before the side effect is executed. useEffect is commonly used for non-critical side effects, such as data fetching, event listeners, or subscriptions.
- useLayoutEffect: Runs synchronously after the component has rendered but before the browser has painted the screen. This blocks the browser from updating the screen, allowing you to perform side effects that require immediate access to the updated DOM. useLayoutEffect is commonly used for side effects that involve measuring or manipulating the DOM, such as calculating element dimensions or performing animations.
Example:
import React, { useEffect, useLayoutEffect } from 'react';
function MyComponent() {
useEffect(() => {
console.log('useEffect');
// Perform non-critical side effects here
});
useLayoutEffect(() => {
console.log('useLayoutEffect');
// Perform side effects that require immediate access to the DOM here
});
return <div>My Component</div>;
}
In the example above, the useEffect hook is used for non-critical side effects, such as logging to the console. The useLayoutEffect hook is used for side effects that require immediate access to the DOM, such as manipulating the layout or measuring element dimensions.
It’s important to note that useLayoutEffect can potentially cause performance issues if the side effects it performs are computationally expensive or cause layout thrashing. Therefore, it’s recommended to use useLayoutEffect sparingly and only when necessary. In most cases, useEffect is sufficient for handling side effects in a React component.
19. How do you handle error boundaries with React Hooks?
With React Hooks, you can handle error boundaries using the useState and useEffect hooks. Here’s how you can implement an error boundary with React Hooks:
Create an ErrorBoundary component: Create a new functional component called ErrorBoundary. Inside this component, use the useState hook to manage a state variable that tracks whether an error has occurred.
import React, { useState } from 'react';
function ErrorBoundary({ children }) {
const [hasError, setHasError] = useState(false);
if (hasError) {
// Render fallback UI when an error occurs
return <div>Something went wrong.</div>;
}
return children;
}
Wrap components with the ErrorBoundary: Wrap the components that you want to be covered by the error boundary with the ErrorBoundary component.
function App() {
return (
<ErrorBoundary>
{/* Render your components here */}
</ErrorBoundary>
);
}
Implement error handling in useEffect: Inside the ErrorBoundary component, use the useEffect hook to handle errors. In the useEffect callback, set up an error boundary by wrapping the code that might throw an error with a try-catch block. If an error occurs, update the state variable to indicate that an error has occurred.
import React, { useState, useEffect } from 'react';
function ErrorBoundary({ children }) {
const [hasError, setHasError] = useState(false);
useEffect(() => {
try {
// Code that might throw an error
} catch (error) {
setHasError(true);
}
}, []);
if (hasError) {
// Render fallback UI when an error occurs
return <div>Something went wrong.</div>;
}
return children;
}
By implementing an error boundary with React Hooks, you can catch and handle errors that occur within the wrapped components. This helps prevent the entire application from crashing and allows you to display a fallback UI or take other appropriate actions when an error occurs.
20. What are the best practices for using React Hooks?
Best practices for using React Hooks:
- Follow the rules of Hooks. These rules are designed to ensure that Hooks work as intended and to avoid bugs.
- Use Hooks for stateful logic. Hooks are best used for managing state within function components.
- Keep Hooks small and focused. This makes them reusable and easier to maintain.
- Use custom Hooks for reusable logic. Custom Hooks allow you to encapsulate reusable logic and share it across multiple components.
- Use dependency arrays correctly. Dependency arrays specify the dependencies that an effect or memoized callback function depends on. This prevents unnecessary re-renders or stale data.
- Optimize performance with useMemo and useCallback. These Hooks memoize expensive computations and callback functions, which can improve performance.
- Test Hooks thoroughly. It’s important to test your Hooks to ensure they work as expected.
- Stay up-to-date with React documentation. React Hooks are still evolving, so it’s important to stay up-to-date with the latest updates and best practices.
- Use Hooks consistently. Once you start using Hooks in a component, try to use them consistently throughout the component. This will make your code more readable and maintainable.
- Use Hooks to abstract away complex logic. Hooks can be used to encapsulate complex logic and provide a clean interface for other components to consume. This can make your code more modular and reusable.
- Don’t be afraid to experiment. Hooks are a powerful tool, but there is no one-size-fits-all approach to using them. Experiment with different ways of using Hooks to find what works best for your codebase.
By following these best practices, you can write clean, maintainable, and performant code when using React Hooks.